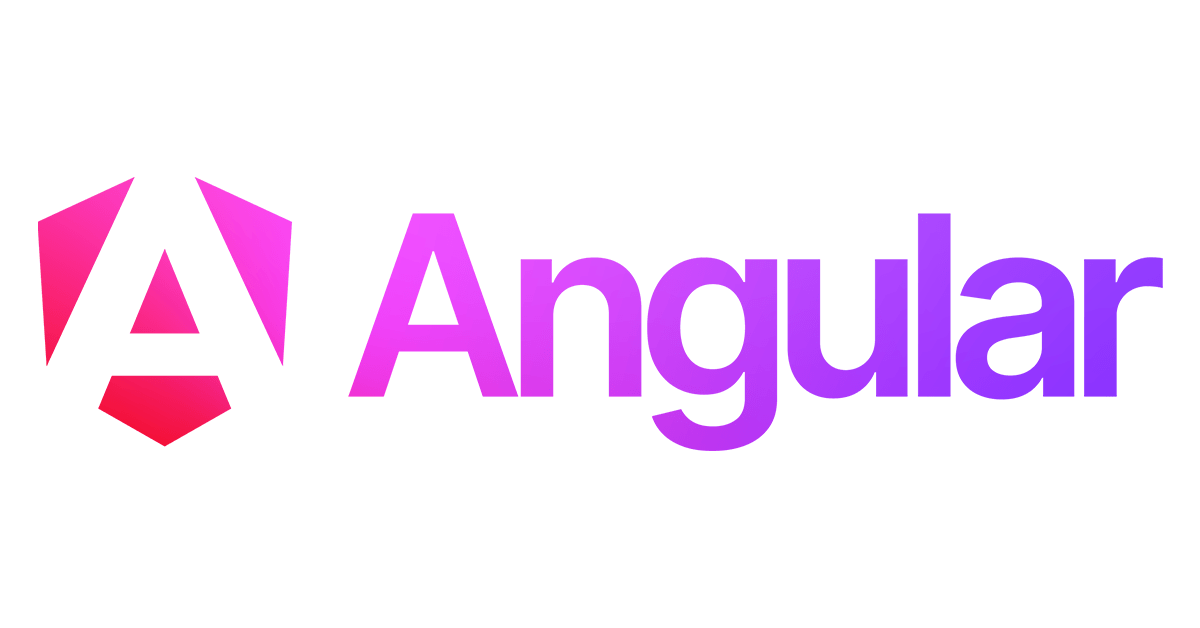
barKoder Barcode Scanner SDK plugin for Angular
How To Utilize the barKoder SDK With Angular
This starter app example showcases the integration of the barKoder Barcode Scanner SDK for Cordova with Angular.
The barKoder Barcode Scanner SDK is an enterprise-grade solution offering a highly customizable interface for barcode scanning in both iOS and Android apps.
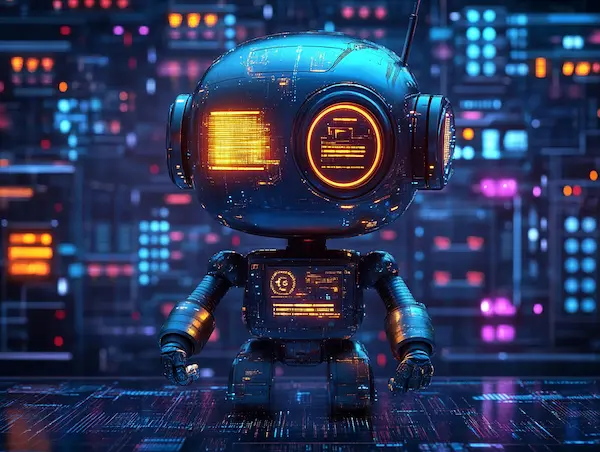
Prerequisites
First, we need to install our Cordova environment. Follow these steps:
Install Cordova
npm install -g cordova
Create a New Cordova Project
cordova create my-cordova-app com.example.cordovaapp CordovaApp
Navigate into the Cordova Project
cd my-cordova-app
Add Platforms
cordova platform add ios
cordova platform add android
Open an IDE and Start Coding
Launch your preferred IDE or text editor and begin building your app using web technologies.
Build and Run
Use Cordova commands to build and run your app on different platforms.
cordova build ios
cordova build android
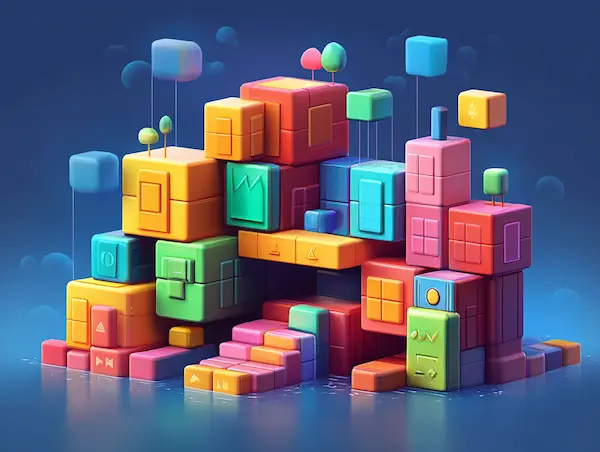
barKoder requirements
Next, we need to install the barKoder Cordova plugin. This can be done by adding it directly from npm, like so:
cordova plugin add barkoder-cordova
Alternatively, for the more adventurous coders who prefer working with local files, you can install it manually from a local folder.
To do this, first download the barKoder plugin from the repository of our Developer Portal. The repository requires an account, so if you don't already have a barKoder account, you'll be asked to create one.
Another option is to use the code from npm—download it and use it locally.
To complete the installation process, follow these steps:
- Download the zip file from the Developer Portal repository
- Extract the contents of the zip file
- Rename the extracted folder as desired (e.g., "barkoder-cordova")
- Move the renamed folder to a location of your choice, but keep it outside the project directory
Finally:
cordova plugin add “/your-path/barkoder-cordova”
Angular setup
With Cordova and barKoder set up, your development environment is now ready for the main task. However, we also want to use Angular in our project. To accomplish this, we need to install the appropriate framework, much like we did earlier:
npm install -g @angular/cli
Then, in your Cordova app folder, run the following command that will create a new Angular application:
ng new angular_app
cd angular_app
Configure your Angular build output for Cordova by updating the angular.json file in your Angular project. Set it to output the build to the www directory used by Cordova.
{
...
"projects": {
"angular_app": {
...
"architect": {
"build": {
"options": {
"outputPath": "../www",
...
},
...
},
...
}
}
}
}
Next, add the cordova.js script to src/index.html
<script src="cordova.js"></script>
Building the Angular App
With all the previous steps completed, we can now begin building Angular components to host our scanner app.
Let's start by generating a new component
ng generate component BarcodeScanner
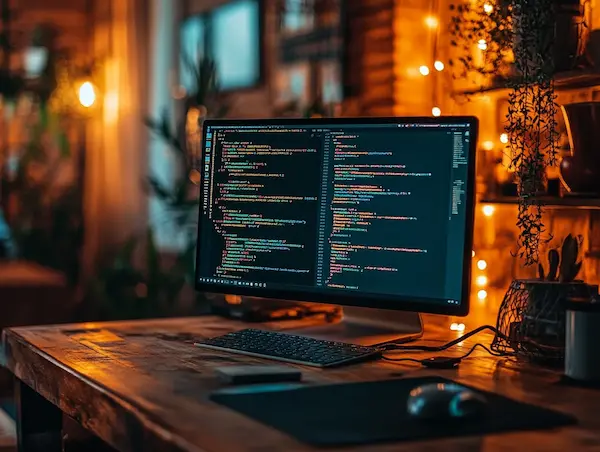
Next, add the following code to your TypeScript file:
declare var Barkoder: any;
import { BarcodeType } from 'plugins/barkoder-cordova-plugin/www/BarkoderConfig';
@ViewChild('barkoderView') barkoderViewRef!: ElementRef;
setActiveBarcodeTypes() {
Barkoder.setBarcodeTypeEnabled(BarcodeType.code128, true);
Barkoder.setBarcodeTypeEnabled(BarcodeType.ean13, true);
}
setBarkoderSettings() {
Barkoder.setRegionOfInterestVisible(true);
Barkoder.setRegionOfInterest(5, 5, 90, 90);
Barkoder.setCloseSessionOnResultEnabled(false);
Barkoder.setImageResultEnabled(true);
Barkoder.setBarcodeThumbnailOnResultEnabled(true);
Barkoder.setBeepOnSuccessEnabled(true);
Barkoder.setPinchToZoomEnabled(true);
Barkoder.setZoomFactor(2.0);
}
async startScanning() {
const boundingRect = this.barkoderViewRef.nativeElement.getBoundingClientRect() as DOMRect;
Barkoder.registerWithLicenseKey("your_license_key");
await Barkoder.initialize(
Math.round(boundingRect.width),
Math.round(boundingRect.height),
Math.round(boundingRect.x),
Math.round(boundingRect.y))
this.setBarkoderSettings();
this.setActiveBarcodeTypes();
Barkoder.startScanning((barkoderResult: any) => {
console.log("Result: " + barkoderResult.textualData);
}, (err: any) => {
console.log(err);
});
}
In your HTML file, add the barkoderView div with an ID:
<div id="barkoderView" #barkoderView >
In your SCSS file, set the desired height for the barkoderView:
#barkoderView {
height: 400px;
}
Licensing
If you're observant, you might have noticed the line Barkoder.registerWithLicenseKey("your_license_key");
within the startScanning
method. This is where you'll input your valid license key, obtainable from our barKoder developer portal.
Running the barKoder Barcode Scanner SDK without a valid trial or production license will result in all successful barcode scan results being partially masked with asterisks (*). Don't worry, though! You can easily get a trial license by registering on the barKoder Portal and using the self-service for generating an evaluation license.
Each trial license is valid for 30 days and can be used on up to 25 devices.
Run the Angular Application
Now it's time to build and run the app we've created. First, let's build the Angular app:
ng build
Finally, run the app on your chosen platform. For Android, use these commands:
cd ..
cordova build android
cordova run android
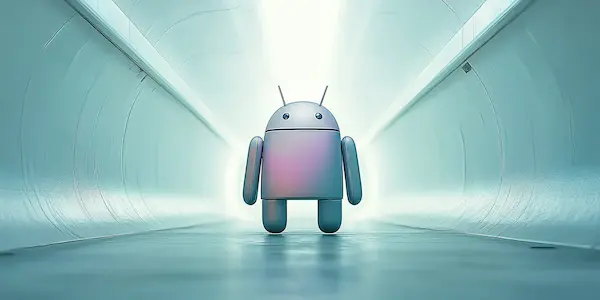
Wrapping Up
At this point, you've successfully created a running Cordova app that integrates barKoder's Cordova plugin with the Angular framework. While this example is intentionally simple, there's ample room for enhancement. Future articles will explore how to enable various barKoder features, significantly expanding the app's capabilities.
Stay tuned for upcoming articles exploring:
For more in-depth information, explore our ever-expanding documentation section.
Found a bug or need clarification? Email us at support@barkoder.com
. Your input helps us enhance our software and documentation.
Happy coding, and may your barcodes always scan true!