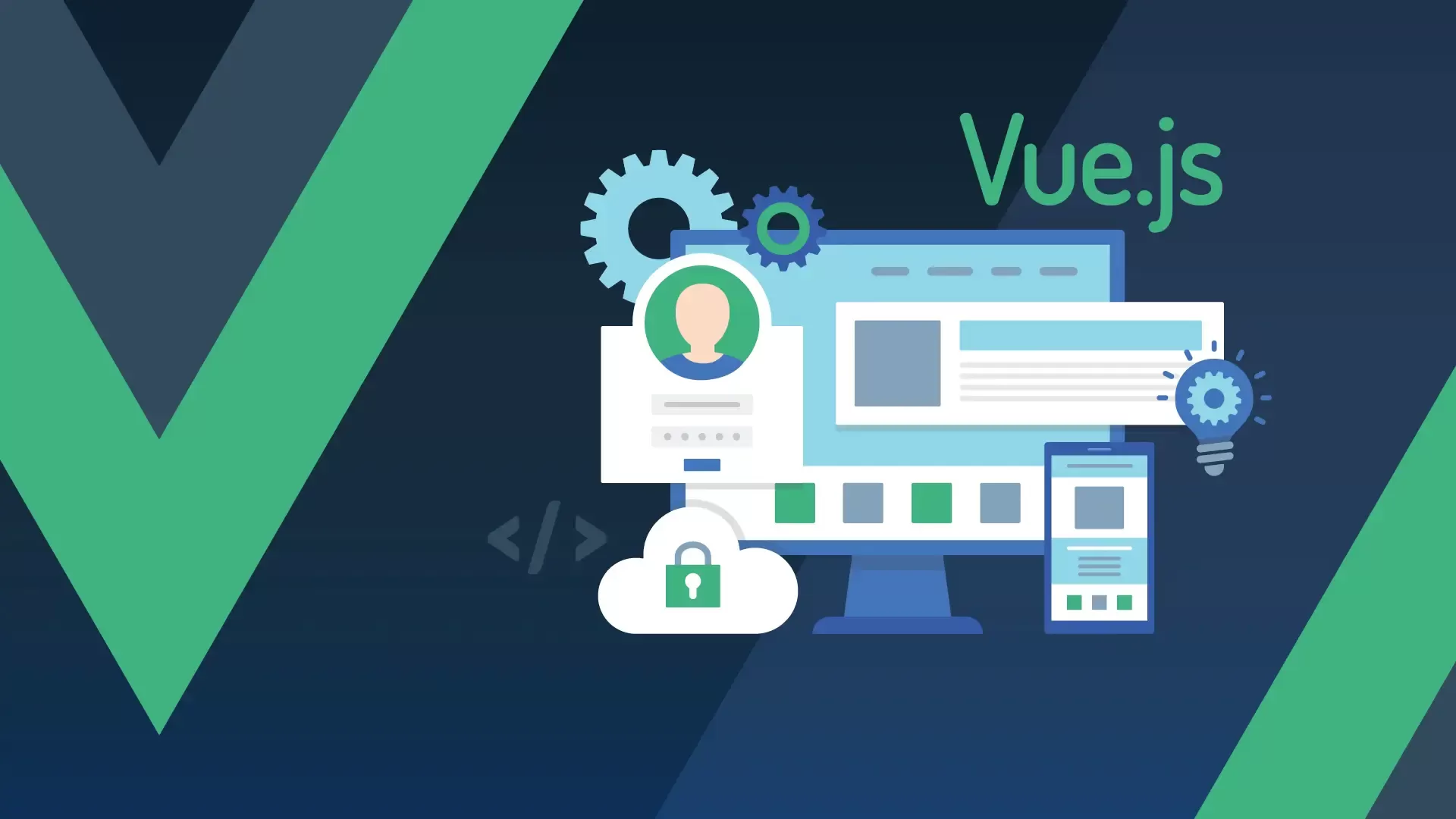
Cordova with Vue Starter Example with barKoder Barcode Scanner SDK
How to use barKoder SDK with VueJS and Cordova
Overview
In the early days of mobile development, life was simpler. Native features on smartphones were accessed through platform-specific SDKs, while web functionality relied on HTML and JavaScript. Then came the era of complexity!
Cross-platform frameworks emerged, making development more intriguing—and, as some might argue, considerably more frustrating. Yet, despite the initial challenges of harmonizing web and native components, time has shown that this complexity can yield remarkably smooth solutions.
This article will try to showcase the integration between Cordova, barKoderSDK and Vue.js
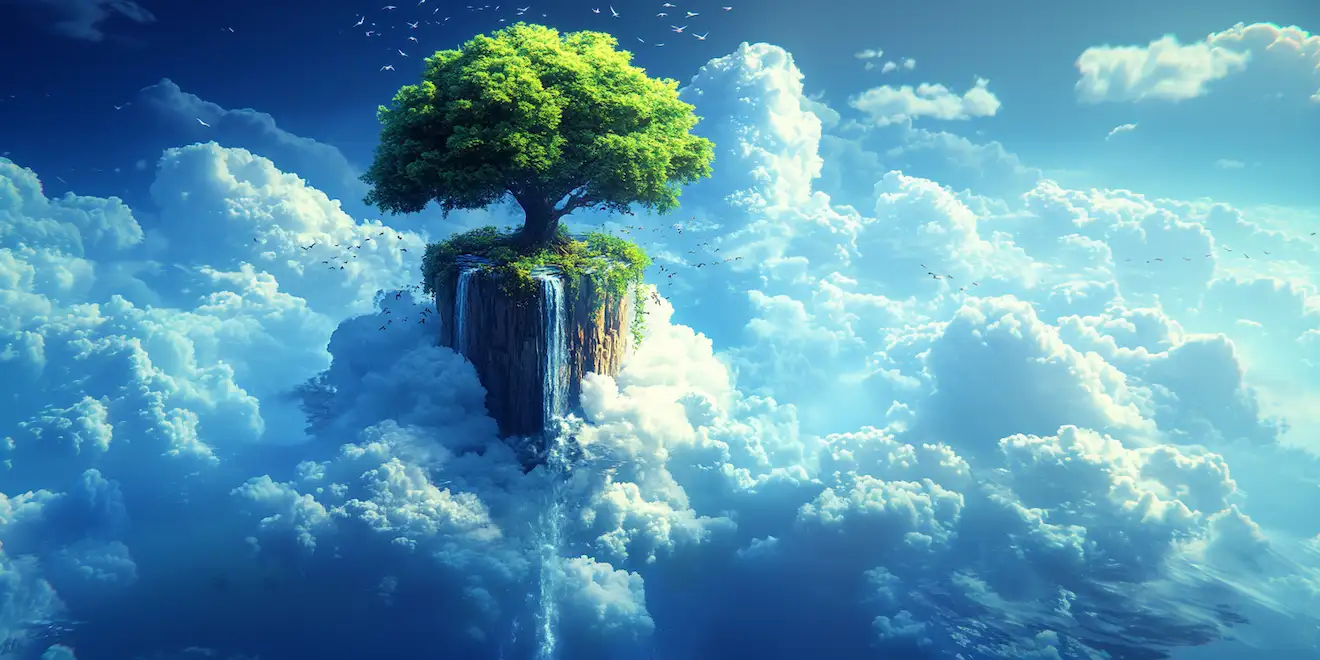
Cordova is considered a pioneering cross-platform solution, and Vue.js is one of the leading JavaScript frameworks. Meanwhile, our very own product, the barKoder Barcode Scanner SDK for Cordova, completes this powerful trio.
The barKoder Barcode Scanner SDK is an enterprise-grade solution that provides a highly customisable interface for barcode scanning in both iOS and Android applications.
Key features of the project
- Uses Vue.js to build the app's user interface.
- Incorporates the barKoder Barcode Scanner SDK for advanced scanning capabilities.
- Uses Cordova to enable smooth cross-platform deployment to iOS and Android.
- Supports a broad range of barcode types, including both 1D and 2D formats.
Prerequisites
Cordova is a cross-platform app runtime that enables developers to build web apps that run natively on iOS, Android, and the Web. As one of the pioneering frameworks, it bridges the gap between web code and native platform features. Despite the emergence of alternatives, Cordova remains a valuable tool and a preferred choice for many project managers seeking a cross-platform solution.
In this project, we will use Cordova to build our app, demonstrating how easily it can be integrated with our SDK and other JavaScript frameworks—specifically Vue.js. To get started, you'll need to ensure the following prerequisites are met:
Node.js and npm
Node.js: Install Node.js version 10 or later on your machine, as required by Cordova.
npm (Node Package Manager): Typically bundled with Node.js, npm will be used to manage dependencies.
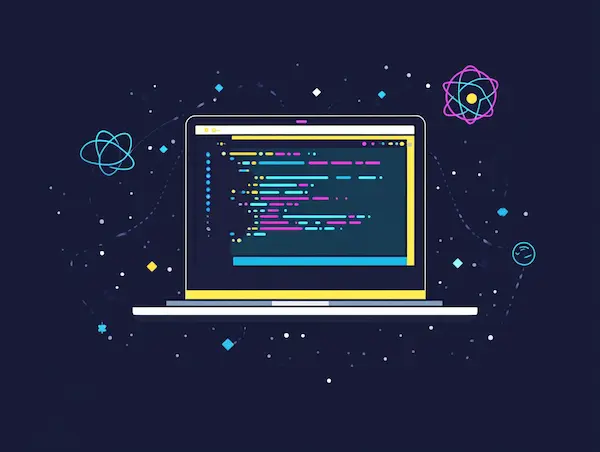
Text Editor or IDE
Choose a text editor or integrated development environment (IDE) for coding. Recommended options include:
Install Git for version control and project management. Git will help you track changes and collaborate with others on the project.
Command Line Interface (CLI)
Ensure you have a command line interface (CLI) installed and accessible, as Cordova commands are executed via the command line. Popular options include:
- Terminal (macOS/Linux)
- Command Prompt (Windows)
- PowerShell (Windows)
iOS Development: Install Xcode (macOS only) for iOS development. Xcode provides the necessary tools and emulators for testing your app on iOS devices.
Android Development: Install Android Studio for Android development. Android Studio includes the Android SDK and an emulator for testing your app on Android devices.
Cordova requirements
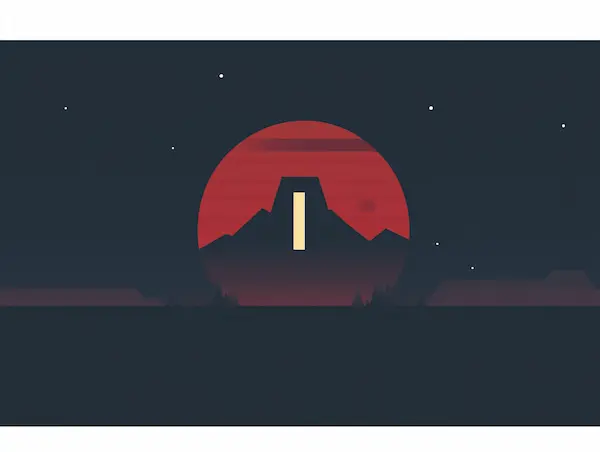
Install Cordova Globally
Install Cordova globally on your machine:
npm install -g cordova
Create a New Cordova Project
To create a new Cordova project, use the Cordova Command Line Interface (CLI). Execute the following command in your terminal or command prompt:
cordova create my-cordova-app com.example.cordovaapp CordovaApp
Navigate to the Cordova Project
Navigate to the newly created Cordova project directory using the command line interface. This step is crucial—we've found it's often unintentionally skipped.
cd my-cordova-app
Add Android Platform
For this example, we'll focus solely on the Android platform. Add the Android platform to your Cordova app:
cordova platform add android
If this app were to run on iOS as well, you could add that platform with
cordova platform add ios
barKoder Barcode Scanner SDK plugin for Cordova
The barKoder Barcode Scanner SDK transforms smartphones and tablets into powerful barcode scanning devices. It features a robust barcode reading engine that supports various barcode types with exceptional speed. barKoder provides a plugin for the Cordova cross-platform framework, allowing us to use these native features within a web app. To implement this functionality, we need to add the plugin to the app we've created so far.
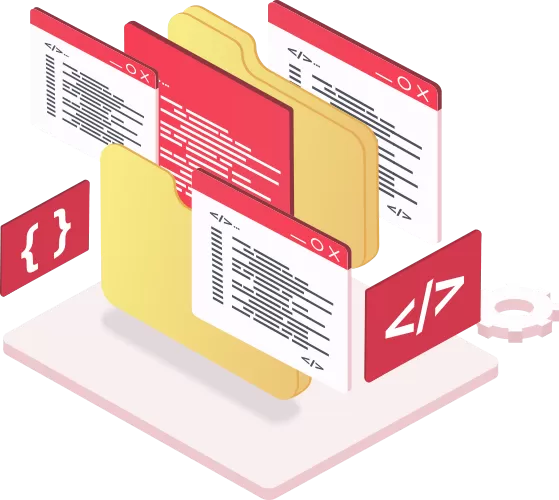
Add the Barkoder-Cordova Plugin
Add the barkoder-cordova
plugin:
cordova plugin add barkoder-cordova
Alternatively, for the more adventurous coders who prefer working with local files, you can install it manually from a local folder.
To do this, first download the barKoder plugin from the Repository within the barKoder Developer Portal. The repository requires an account, so if you don't already have a barKoder account, you'll be asked to create one.
Another option is to use the code from npm—download it and use it locally.
To complete the installation process, follow these steps:
- Download the zip file from the Repository page of the Developer Portal
- Extract the contents of the zip file
- Rename the extracted folder as desired (e.g., "barkoder-cordova")
- Move the renamed folder to a location of your choice, but keep it outside the project directory
Finally:
cordova plugin add “/your-path/barkoder-cordova”
Vue.js Setup
Vue.js is a progressive JavaScript framework for building user interfaces. It's designed to be incrementally adoptable, allowing developers to integrate it into existing projects easily or use it as a full-featured framework for building complex applications.
Now that we have Cordova (https://cordova.apache.org/) and barKoder (https://github.com/barKoderSDK/barkoder-cordova-plugin) installed, it's time to add Vue.js to the project. Here are the steps to integrate Vue.js:
Install Vue.js inside the Cordova project:
npm install -g @vue/cli
Create a new Vue.js application:
vue create vue_app
Configure Vue.js build output for Cordova:
Update the Vue.js project's build configuration to output to the www
directory used by Cordova. Edit vue.config.js
in your Vue.js project to set the output directory:
module.exports = {
outputDir: '../www',
// other configurations as needed
};
Build Vue.js Application
Build the Vue.js application:
cd vue_app
npm run build
Ensure cordova.js
is included
Make sure the cordova.js
script is included in public/index.html
:
<script src="cordova.js"></script>
Test your app
Navigate back to the Cordova project root and test or run your app. You will do this again (or multiple more times) once the main code of the app is up and running, this time however we want to make sure that everything is setup properly
cd ..
cordova build android
cordova run android or cordova run ios
You should now have successfully built the Vue app. This step is crucial for the rest of the code to work correctly. If you've run into any problems, email us at support@barkoder.com
for help.
Vue.js Components
Next, let’s start building the app. In your component/[fileName].vue add:
<template>
<div id="barkoderView" ref="barkoderView"></div>
</template>
<script>
import { ref, onMounted } from 'vue';
import { BarcodeType } from '../../../plugins/barkoder-cordova-plugin/www/BarkoderConfig.js';
export default {
setup() {
const barkoderViewRef = ref('');
const scannedResult = ref(null);
const isScanning = ref(false);
const setActiveBarcodeTypes = async () => {
try {
await window.Barkoder.setBarcodeTypeEnabled(BarcodeType.code128, true );
await window.Barkoder.setBarcodeTypeEnabled(BarcodeType.ean13, true);
} catch (error) {
console.error('Error setting active barcode types:', error);
throw error;
}
};
const setBarkoderSettings = async () => {
try {
window.Barkoder.setRegionOfInterestVisible(true);
window.Barkoder.setRegionOfInterest(5, 5, 90, 90);
window.Barkoder.setCloseSessionOnResultEnabled( false );
window.Barkoder.setImageResultEnabled( true );
window.Barkoder.setBarcodeThumbnailOnResultEnabled( true );
window.Barkoder.setBeepOnSuccessEnabled( true );
window.Barkoder.setPinchToZoomEnabled( true );
window.Barkoder.setZoomFactor( 2.0 );
} catch (error) {
console.error('Error setting Barkoder settings:', error);
throw error;
}
};
const startScanning = async () => {
scannedResult.value = {
textualData: null,
type: null,
thumbnailImage: null,
};
isScanning.value = true;
try {
const boundingRect = await barkoderViewRef.value.getBoundingClientRect();
window.Barkoder.registerWithLicenseKey('your_license_key');
await new Promise((resolve, reject) => {
window.Barkoder.initialize(
Math.round(boundingRect.width),
Math.round(boundingRect.height),
Math.round(boundingRect.x),
Math.round(boundingRect.y),
() => {
resolve();
},
(error) => {
reject('Initialization error: ' + error);
}
);
});
await setBarkoderSettings();
await setActiveBarcodeTypes();
document.addEventListener('deviceready', () => {
if (window.Barkoder) {
window.Barkoder.startScanning(
(barkoderResult) => {
scannedResult.value = {
textualData: barkoderResult.textualData,
type: barkoderResult.barcodeTypeName,
resultImage: "data:image/jpeg;base64," + barkoderResult.resultImageAsBase64,
thumbnailImage: "data:image/jpeg;base64," + barkoderResult.resultThumbnailAsBase64,
};
window.Barkoder.stopScanning();
isScanning.value = false;
},
(error) => {
console.error('Scanning error:', error);
}
);
} else {
console.error('BarkoderScanner plugin not available');
}
}, false);
} catch (error) {
alert('Error: ' + error);
}
};
const stopScanning = () => {
document.addEventListener('deviceready', () => {
if (window.Barkoder) {
window.Barkoder.stopScanning(
() => isScanning.value = false,
(error) => console.error('Stop scanning error:', error)
);
} else {
console.error('BarkoderScanner plugin not available');
}
}, false);
};
onMounted(() => {
barkoderViewRef.value = document.getElementById('barkoderView');
});
return {
barkoderViewRef,
startScanning,
stopScanning,
scannedResult,
isScanning
};
}
};
</script>
In your SCSS file, set the desired height for the barkoderView:
#barkoderView {
height: 400px;
}
Considerations
- If you cannot read from BarkoderConfig.ts Create a file named BarkoderConfig.js in the plugins/barkoder-cordova-plugin/www/ directory. Copy the contents of BarkoderConfig.ts into this new file and use it directly as a normal JavaScript file.
Licensing
If you're observant, you might have noticed the line window.Barkoder.registerWithLicenseKey('your_license_key');
within the startScanning
method. This is where you'll input your valid license key, obtainable from our barKoder developer portal.
Running the barKoder Barcode Scanner SDK without a valid trial or production license will result in all successful barcode scan results being partially masked with asterisks (*). Don't worry, though! You can easily get a trial license by registering on the barKoder Portal and using the self-service for generating a trial license.
Each trial license is valid for 30 days and can be used on up to 25 devices.
Wrapping up
_391907904.webp)
In the end, we've added a bit of complexity, but many would argue it's worth it. These small complications often lead to significant progress. The final product is a functional Cordova application that seamlessly integrates barKoder's Cordova plugin with the Vue.js framework. While this example serves as an excellent starting point, it offers substantial potential for further development. In upcoming articles, we'll explore how to enable various advanced barKoder features, which will significantly enhance your application's functionality and versatility.
Stay tuned for upcoming articles exploring:
_1351163186.webp)
1D and 2D Templates
For more in-depth information, explore our comprehensive documentation. If you encounter any issues or need clarification, please email us at support@barkoder.com. Your feedback is invaluable in helping us improve our software and documentation.