Installation Guide for barKoder's iOS Barcode Scanner SDK
Please follow these simple steps to integrate our SDK into your iOS project.
Step 1: Create a new group #
Name the group “frameworks”. This name is optional, you can use any label you prefer.
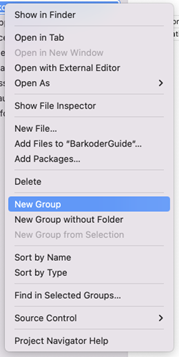
Step 2: Add Barkoder.xcframework and BarkoderSDK.xcframework #
Add these items to the frameworks group (Copy items if needed, Create groups and Add to desired targets).
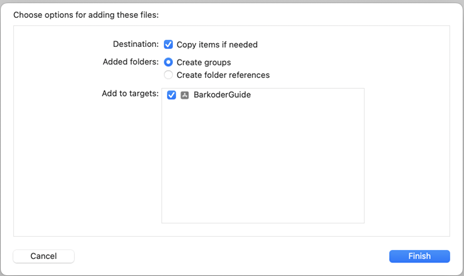
Step 3: Enable Bitcode (set it to NO) #
In Build Settings, set Enable Bitcode to NO as shown in the image.
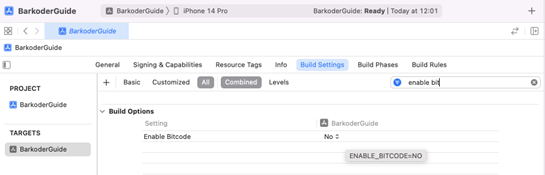
Step 4: Update Info.plist #
Open Info.plist with Source Code and add the following changes:
<key>NSCameraUsageDescription</key>
<string>BKD Scanner requires using camera</string>
Or using Property List add “Privacy - Camera Usage Description” and key "BKD Scanner requires using camera”

Step 5: Add BarkoderView as a view #
Import BarkoderSDK and delcare barkoderView as a view
import UIKit
import BarkoderSDK
class ViewController: UIViewController {
private var barkoderView: BarkoderView!
override func viewDidLoad() {
super.viewDidLoad()
setupUI()
setupConstraints()
}
private func setupUI() {
barkoderView = BarkoderView()
view.addSubview(barkoderView)
}
private func setupConstraints() {
barkoderView.translatesAutoresizingMaskIntoConstraints = false
barkoderView.leadingAnchor.constraint(equalTo: view.leadingAnchor).isActive = true
barkoderView.topAnchor.constraint(equalTo: view.topAnchor).isActive = true
barkoderView.trailingAnchor.constraint(equalTo: view.trailingAnchor).isActive = true
barkoderView.bottomAnchor.constraint(equalTo: view.bottomAnchor).isActive = true
}
}
Step 6: Licensing #
The SDK will scan barcodes even without a license, but certain characters will be marked with asterisks. To prevent this, you can create a trial license or a production license. Follow barkoder.com/register to create a trial license today.
Step 7: Create Barkoder Config #
Setup the Barkoder Config according to your needs:
To perform scanning, the config property must be properly set.
As mentioned, if the license key is not valid, you will receive results with asterisks inside.
private func createBarkoderConfig() {
barkoderView.config = BarkoderConfig(licenseKey: "LICENSE_KEY") { licenseResult in print("Licensing SDK: \(licenseResult)") }
// Enable QR barcode type
guard let decoderConfig = barkoderView.config?.decoderConfig else { return }
decoderConfig.qr.enabled = true
}
Step 8: Implement BarkoderResultDelegate protocol #
To receive the scanned results we need to implement this protocol:
extension ViewController: BarkoderResultDelegate {
func scanningFinished(_ decoderResults: [DecoderResult], thumbnails: [UIImage]?, image: UIImage?) {
if let textualData = decoderResults[0].textualData {
print("Scanned result: ", textualData)
}
}
}
Step 9: Start the scanning process #
And finally start the scanning process:
try? barkoderView.startScanning(self)
For further assistance, you can submit a ticket through the Issue System of the barKoder Developer Portal. Don't hesitate to request a quote if you have any questions or need additional support.